INHERITANCE
Inheritance is the concept by which the properties of one entity are available to another. In C++, inheritance is the property by which the objects of a derived class possess copies of the data members and member functions of the base class.
A class that inherits or derives attributes from another class is called a derived class or sub class and the class from which it is derived is called a base class or super class. Each instance of a derived class includes most of the attributes of the base class. Hence, a derived class has a larger set of attributes than its base class.
Any class can be a base class. More than one class can inherit attributes from a single base class, and a derived class can be a base class to another class.
Syn:
class <base_class>
{
. . . . . . . . . . .
. . . . . . . . . . .
};
class <derived_class : <access_specifier> <base_class>
{
. . . . . . . . . . .
. . . . . . . . . . .
}
In the above declaration, the single colon ( : ) is used to specify that the class being declared is derived from another class (the name of which is specified after the colon).
Access specifier/Qualifier:
• private : If the members of a class declared as private, can not be accessed from its sub- classes and other classes.
• public : If the members of a class declared as public, can be accessed from its sub-class as well as from other classes also.
• protected : If the members of a class declared as protected can be accessed from the derived classes, but cannot be accessed from anywhere in other classes.
If the private qualifier is used when deriving a class, all
private members of base class becomes private in derived class.
public members of base class becomes private in derived class.
protected members of base class becomes private in derived class.
If the public qualifier is used when deriving a class, all
private members of base class becomes private in derived class.
public members of base class becomes public in derived class.
protected members of base class becomes protected in derived class.
If the protected qualifier is used when deriving a class, all
private members of base class becomes private in derived class.
public members of base class becomes protected in derived class.
protected members of base class becomes protected in derived class.
TYPES OF INHERITANCE:
1. Single Inheritance
2. Multiple Inheritance
3. Multi-level Inheritance
SINGLE INHERITANCE:
If the derived class inherits the properties from one base class , is called a single inheritance.
Consider, parent having the properties in Base class and inherited to Derived class. Now, Derived class having the properties of Base class and properties of child, which is exist already in derived class.
// Ex-22: PGM ILLUSTRATES SINGLE INHERITANCE
#include<conio.h>
#include<iostream.h>
class parent
{
private:
int a,b;
public:
void set()
{
a=30;
b=60;
}
void get()
{
cout<<" "<<a<<" "<<b<<" ";
}
};
class child : public parent
{
private:
int c;
public:
void set1()
{
c=90;
}
void get1()
{
cout<<c;
}
};
void main()
{
clrscr();
child ob;
ob.set();
ob.set1();
ob.get();
ob.get1();
getch();
}
#include<iostream.h>
class parent
{
private:
int a,b;
public:
void set()
{
a=30;
b=60;
}
void get()
{
cout<<" "<<a<<" "<<b<<" ";
}
};
class child : public parent
{
private:
int c;
public:
void set1()
{
c=90;
}
void get1()
{
cout<<c;
}
};
void main()
{
clrscr();
child ob;
ob.set();
ob.set1();
ob.get();
ob.get1();
getch();
}
// Ex-23: PGM ILLUSTRATES SINGLE ILLUSTRATES (PASSING VALUE THROUGH AN OBJECT)
#include<conio.h>
#include<iostream.h>
class parent
{
protected:
int x;
};
class child : public parent
{
private:
int y;
public:
void set(int a, int b)
{
x=a;
y=b;
}
void get()
{
cout<<"x= "<<x<<"\ny="<<y;
}
};
void main()
{
clrscr();
child ob;
ob.set(5,10);
ob.get();
getch();
}
#include<iostream.h>
class parent
{
protected:
int x;
};
class child : public parent
{
private:
int y;
public:
void set(int a, int b)
{
x=a;
y=b;
}
void get()
{
cout<<"x= "<<x<<"\ny="<<y;
}
};
void main()
{
clrscr();
child ob;
ob.set(5,10);
ob.get();
getch();
}
MULTIPLE INHERITANCE:
If the derived class inherits the properties from more than one base class, is called multiple inheritance.
// Ex-24: PGM ILLUSTRATES MULTIPLE INHERITANCE
#include<iostream.h>
#include<conio.h>
#include<string.h>
class father
{
private:
char fname[20];
public:
void set1(char* name1)
{
strcpy(fname,name1);
}
void get1()
{
cout<<"Father name = "<<fname<<endl;
}
};
class mother
{
private:
char mname[20];
public:
void set2(char* name2)
{
strcpy(mname,name2);
}
void get2()
{
cout<<"Mother name = "<<mname<<endl;
}
};
class son : public father, public mother
{
private:
char sname[20];
public:
void set3(char* name3)
{
strcpy(sname,name3);
}
void get3()
{
cout<<"Son name = "<<sname<<endl;
}
};
void main(void)
{
son object;
object.set1("Rathinavel");
object.set2("Meena");
object.set3("Kishore");
clrscr();
object.get1();
object.get2();
object.get3();
getch();
}
#include<conio.h>
#include<string.h>
class father
{
private:
char fname[20];
public:
void set1(char* name1)
{
strcpy(fname,name1);
}
void get1()
{
cout<<"Father name = "<<fname<<endl;
}
};
class mother
{
private:
char mname[20];
public:
void set2(char* name2)
{
strcpy(mname,name2);
}
void get2()
{
cout<<"Mother name = "<<mname<<endl;
}
};
class son : public father, public mother
{
private:
char sname[20];
public:
void set3(char* name3)
{
strcpy(sname,name3);
}
void get3()
{
cout<<"Son name = "<<sname<<endl;
}
};
void main(void)
{
son object;
object.set1("Rathinavel");
object.set2("Meena");
object.set3("Kishore");
clrscr();
object.get1();
object.get2();
object.get3();
getch();
}
MULTILEVEL INHERITANCE:
The properties of Base class(Grandfa) are inherited to derived class(father), which can consider as base class to another derived class(son). The following diagram shows this.
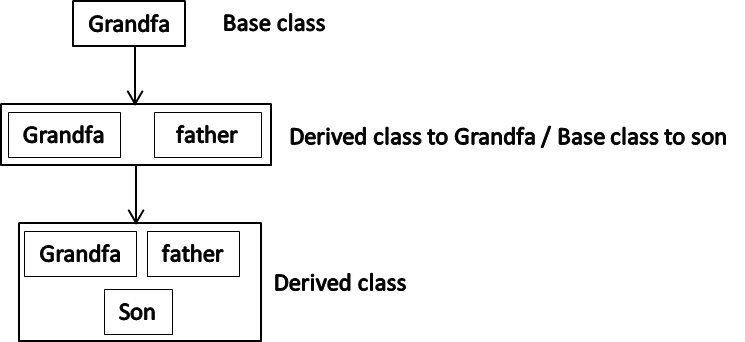
In above diagram, the base class Grandfa having some properties and derived to the derived class father. Then the properties of both Grandfa and father, derived to the class son.
So, Grandfa is a direct base class to the derived class father and indirect base class to the derived class son. Also the
class father is a direct base class to the derived class son.
Therefore, the class son having the properties of Grandfa, father and it’s own.
// Ex-25: PGM ILLUSTRATES MULTILEVEL INHERITANCE
#include<iostream.h>
#include<conio.h>
class Grandfa
{
protected:
int p;
};
class father : public Grandfa
{
private:
int q;
public:
void setfather(int b)
{ q=b; }
void getfather()
{ cout<<"father = "<<q<<endl; }
};
class son : public father
{
private:
int r;
public:
void setson(int a, int c)
{
p=a;
r=c;
}
void getson()
{
cout<<"Son = "<<r<<endl<<"Grandfa = "<<p<<endl;
}
};
void main(void)
{
son ob;
ob.setson(75,25);
ob.setfather(50);
clrscr();
ob.getson();
ob.getfather();
getch();
}
#include<conio.h>
class Grandfa
{
protected:
int p;
};
class father : public Grandfa
{
private:
int q;
public:
void setfather(int b)
{ q=b; }
void getfather()
{ cout<<"father = "<<q<<endl; }
};
class son : public father
{
private:
int r;
public:
void setson(int a, int c)
{
p=a;
r=c;
}
void getson()
{
cout<<"Son = "<<r<<endl<<"Grandfa = "<<p<<endl;
}
};
void main(void)
{
son ob;
ob.setson(75,25);
ob.setfather(50);
clrscr();
ob.getson();
ob.getfather();
getch();
}
FRIEND FUNCTION:
When using two functions for accepting data and displaying it in Base class, we would be the overhead involved in making function calls each time the input is accepted from the user and displayed. To reduce the number of unnecessary function calls would be to make the member data itself public. But this would defeat the whole purpose of encapsulation. The answer to this problem is a friend function. A function declared as a friend function of the class acts like just that a friend of the class. It is not a member of the class but it can access all public, protected and private members of the class. A friend function can be declared within the class declaration as follows.
friend void get(void);
Once this is done, the body of the function can be defined anywhere in the program. This function acts as a member function with respect to the class (i.e., it can access all private members) and as an ordinary function with respect to the rest of the program (i.e., it need not be referenced through an object though it is declared as part of the class).
Note:
A friend function can be either a global function or can be a member function of another class. Since a friend function is not part of the class, it can be declared anywhere in the private, protected and public section of the class without its visibility getting affected.
// Ex-26: A CLASS IS FRIENDSHIP TO MEMBER FUNCTION
#include<iostream.h>
#include<conio.h>
class demo
{
private:
int x;
public:
void set(int a);
friend void get(demo ob);
//Here class demo is friendship to member function get()
};
void demo :: set(int a)
{ x = a; }
void get(demo ob)
{ cout<<"x = "<<ob.x<<endl; }
void main()
{
demo obj;
obj.set(50);
clrscr();
get(obj);
getch();
}
#include<conio.h>
class demo
{
private:
int x;
public:
void set(int a);
friend void get(demo ob);
//Here class demo is friendship to member function get()
};
void demo :: set(int a)
{ x = a; }
void get(demo ob)
{ cout<<"x = "<<ob.x<<endl; }
void main()
{
demo obj;
obj.set(50);
clrscr();
get(obj);
getch();
}
// Ex-27: A CLASS IS FRIENDSHIP TO ANOTHER CLASS
#include<conio.h>
#include<iostream.h>
class one
{
friend class two;
private:
int x;
public:
void set(int a)
{ x = a; }
};
class two
{
public:
void get(one ob)
{ cout<<"x = "<<ob.x<<endl; }
};
void main(void)
{
one ob1;
two ob2;
ob1.set(75);
clrscr();
ob2.get(ob1);
getch();
}
#include<iostream.h>
class one
{
friend class two;
private:
int x;
public:
void set(int a)
{ x = a; }
};
class two
{
public:
void get(one ob)
{ cout<<"x = "<<ob.x<<endl; }
};
void main(void)
{
one ob1;
two ob2;
ob1.set(75);
clrscr();
ob2.get(ob1);
getch();
}